Introduction
Entity Framework is an Object Relational Mapper (ORM) from Microsoft that lets the application's developers work with relational data as business models. It eliminates the need for most of the plumbing code that developers write (while using ADO.NET) for data access. Entity Framework provides a comprehensive, model-based system that makes the creation of a data access layer very easy for the developers by freeing them from writing similar data access code for all the domain models. The initial release of Entity Framework was Entity Framework 3.5. It was released with .NET Framework 3.5 SP1 and Visual Studio 2008 SP1. Entity Framework has evolved a lot since then, and the current version is 6.0. Entity Framework eases the task of creating a data access layer by enabling the access of data, by representing the data as a conceptual model, that is, a set of entities and relationships. The application can perform the basic CRUD (create, read, update, and delete) operations and easily manage one-to-one, one-to-many, and many-to-many relationships between the entities. Here are a few benefits of using Entity Framework: • The development time is reduced since the developers don't have to write all the ADO.NET plumbing code needed for data access • We can have all the data access logic written in a higher-level language such as C# rather than writing SQL queries and stored procedures • Since the database tables cannot have advanced relationships (inheritance) as the domain entities can, the business model, that is, the conceptual model can be used to suit the application domain using relationships among the entities. Introduction to Entity Framework [ 8 ] • The underlying data store can be replaced relatively easily if we use an ORM since all the data access logic is present in our application instead of the data layer. If an ORM is not being used, it would be comparatively difficult to do so.
You are going to learn EntityFramework from scratch in this section. We will use EntityFramework 5.0 with Visual Express 2013 for all the basic tutorials.
Versions history of EntityFramework:
EntityFramework Version | Introduced Features |
---|---|
EF 3.5 | Basic O/RM support with Database First approach. |
EF 4.0 | POCO Support, Lazy loading, testability improvements, customizable code generation and the Model First approach. |
EF 4.1 | First to available of NuGet package, Simplified DBContext API over ObjectContext, Code First approach. EF 4.1.1 patch released with bug fixing of 4.1. |
EF 4.3 | Code First Migrations feature that allows a database created by Code First to be incrementally changed as your Code First model evolves. EF 4.3.1 patch released with bug fixing of EF 4.3. |
EF 5.0 | Announced EF as Open Source. Introduced Enum support, table-valued functions, spatial data types, multiple-diagrams per model, coloring of shapes on the design surface and batch import of stored procedures, EF Power Tools and various performance improvements. |
EF 6.0 - Current release | EF 6.0\6.1 is latest release of Entity Framework. It includes many new features related to Code First & EF designer like asynchronous query & save, connection Resiliency, dependency resolution etc. |
Prerequisites: Basic knowledge of .Net Framework, C#, Visual Studio 2008/2010 and MS SQL Server is required.
Audience: Basic tutorials are helpful for anyone who doesn't know anything about Entity Framework and would like to learn it from scratch.
What is Entity Framework?
Entity framework is an Object/Relational Mapping (O/RM) framework. It is an enhancement to ADO.NET that gives developers an automated mechanism for accessing & storing the data in the database, and for working with the results, in addition to DataReader and DataSet.
What is O/RM framework and why do we need it?
ORM is a tool for storing data from domain objects to relational database like MS SQL Server, in an automated way, without much programming. O/RM includes three main parts: Domain class objects, Relational database objects and Mapping information on how domain objects map to relational database objects (tables, views & storedprocedures). ORM allows us to keep our database design separate from our domain class design. This makes the application maintainable and extendable. It also automates standard CRUD operation (Create, Read, Update & Delete) so that the developer doesn't need to write it manually.
There are many ORM frameworks for .net in the market like DataObjects.Net, NHibernate, OpenAccess, SubSonic etc. Entity Framework is an open source ORM framework from Microsoft.
When Entity Framework (EF) 3.5 was first released in 2008, I cried out ‘What the heck’, just like many other developers did across the world. As misunderstood as this technology was, some found it buggy while others felt it was complex. There was also a ‘natural’ resistance from the developer community, especially the ones working on LINQ To SQL a.k.a. Linq2Sql. They simply could not find a good reason for using EF 3.5 over LINQ to SQL.
What is Entity Framework 4.0? Why do we need a new Data Access Technology?
All these years, Microsoft has been providing different ways to communicate with the Database. Developers have been switching from one data access technology to the other, starting with DAO (Data Access Objects), then RDO (Remote Data Objects), then ADO (ActiveX Data Objects) and then finally ADO.NET. ADO.NET has been around from quite some time and Microsoft has invested a great deal of resources in it, with new features added to it every now and then. Although working with the DataSet and DataReader has served our data access needs for many years, a developer spends a lot of time being concerned with the details of the schema, trying to keep up with database schema changes and doing redundant tasks while interacting with the database, over and over again.
The ADO.NET Entity Framework (EF) is an Object/Relational mapping (ORM) framework and is a set of technologies in ADO.NET, for developing applications that interacts with data. We just discussed that an ADO.NET developer spends a lot of time keeping up with the database changes. Well EF provides a mapping from the relational database schema to the objects and offers an abstraction of ADO.NET. So with EF, you can define Entity classes that are independent of a database structure and then map them to the tables and associations of the database. Since we are now working with Entities which have their own schema, we are shielded from the changes in the database. The object context keeps tabs on the entities that are changed.
In simple words, with the Entity Framework, you are architecting, designing and developing at a conceptual level. You are no more worried about the ‘specific details’ of communicating with the database and switching from one relational database to the other is also possible with EF, without much efforts.
What happens to ADO.NET now?
ADO.NET is here to stay! EF was not released with the intension to replace ADO.NET. It is in fact an enhancement to ADO.NET and helps eliminate the gap between the application and the database. Behind the scene, EF uses ADO.NET classes, but the details are abstracted from you. EF provides a shift from Database-oriented (DataReader, DataSet) to Model-oriented development. So instead of focusing on a Database, a developer focuses on the Entity that represents the Business Model of the application.
If the purpose of EF is not yet clear, it will be, in the forthcoming articles when we will explore its features and look at it in detail. Continue reading as I am about to explain why EF was chosen over LINQ To SQL!
When was Entity Framework Released?
Although announced in TechEd 2006, the first version of Entity Framework 3.5 was released two years later in August 2008, along with Visual Studio 2008 SP1 and .NET 3.5 SP1
The second version (also the current version) of Entity Framework i.e. 4.0 was released in April 2010 along with VS 2010 and .NET 4.0.
What happened to LINQ To SQL?
When .NET 3.5 and Visual Studio 2008 was being released in November 2007, a lot of focus was on LINQ. The original plan was to release EF along with VS 2008/.NET 3.5 and introduce developers to this new LINQ based data access technology. However the scale of EF caused a delay and it could not be released along with VS 2008.
Meanwhile, LINQ To SQL (L2S), worked upon by a different team at Microsoft was ready to release. Since one of the focuses of the VS 2008 and .NET 3.5 release was on LINQ, it made a lot of sense to release a LINQ Provider along with VS 2008. So during that time, L2S became the original LINQ provider for data access. In my honest opinion, L2S was kind of a bait to attract developers towards LINQ data access, while the team continued working on EF, i.e. the real beast.
Finally when EF 3.5 was released in August 2008, it could not get the attention from the developer community, for obvious reasons. L2S was gaining popularity primarily due to it being lightweight, simple and easy to use technology and the fact that most of the .NET apps targeted SQL Server, there was a resistance to adopt a new framework (EF) from the dev community. EF remained a misunderstood technology, there were issues in the framework and although accidental, L2S was still believed by many as the original LINQ provider for data access. EF had a lot to prove!
However with the release of EF 4.0 with .NET 4.0, things have changed, including opinions. Microsoft made it clear that although L2S was supported, it was not the recommended technology. Moreover with the new features like POCO support, Model First Development and Custom Code Generation, developers started looking at EF 4.0 and consider it seriously as the preferred ORM solution for enterprise apps.
LINQ To SQL Vs Entity Framework
There are some fundamental differences between L2S and EF which every developer should understand. Both EF and L2S provide a framework for managing relational data as objects, with the objective of programming against a conceptual model rather than a DB schema.
However LINQ To SQL supports rapid development of applications that query only SQL Server and SQL Server Compact 3.5 databases, providing a 1:1 mapping of your existing Database schema to classes. It does not provide the flexibility to use objects that do not exactly match the tables.
EF on the other hand, supports advanced modeling features and ‘loosely coupled and flexible’ mapping of objects to SQL Server. Through extended ADO.NET Data Providers, EF supports other relational databases as well, including Oracle, DB2, MySql etc. EF also allows objects to have a different structure from your database schema.
Although LINQ To SQL (L2S) is supported by Microsoft, it is not recommended. Entity Framework (EF) is definitely the way to go if you are working on something bigger (enterprise apps), need the flexibility of a solid framework, with the support for multiple databases and much more!
Note: LINQ to Entities is part of the Entity Framework and exposes many of the same features as L2S. It has replaced L2S as the standard mechanism for using LINQ on databases.
How do I get Started with Entity Framework 4.0?
Well if you have continued reading so far, I assume you are interested in Entity Framework 4.0 and are ready to explore the future of data access. The easiest way to get started with it is to download and install Visual Studio 2010.
ADO .NET Entity Framework (EF) is an addition to the Microsoft ADO.NET family. It enables developers to create data access applications by programming against a conceptual application model instead of programming directly against a relational storage schema. The goal is to decrease the amount of code and maintenance required for the data-oriented applications. The Entity Framework applications provide the following benefits:
- Applications can work in terms of a more application-centric conceptual model including types with inheritance, complex members, and relationships
- Applications are freed from hard coded dependencies on a particular data engine or storage schema Mappings between the conceptual model and the storage-specific schema can change without changing the application code Developers can work with a consistent application object model that can be mapped to various storage schema s, possibly implemented in different database management systems
- Multiple conceptual models can be mapped to a single storage schema The Language Integrated Query (LINQ) support provides compile-time syntax validation for queries against a conceptual model
With Entity Framework, developers work with a conceptual data model, an Entity Data Model (EDM), instead of the underlying databases.
The conceptual data model schema is expressed in the Conceptual Schema Definition Language (CSDL),
the actual storage model is expressed in the Storage Schema Definition Language (SSDL),
;and the mapping in between is expressed in the Mapping Schema Language (MSL).
A new data-access provider, EntityClient is created for this new framework, but under the hood, the ADO.NET data providers are still being used for communicating with the databases.
OVERHEAD OF USING EF VS ADO.NET
- The overhead of taking a ado.net data reader an transforming the results to objects, and the overhead of compiling SQL queries from LINQ.
You will have the same overhead and even more, if you try to build your own ORM framework. Better don't do it - When you have abstraction layer you get overhead. The overhead depends on what they do with Entity Framework.
- in general you should consider to get away from Inheritance because it does have a greater overhead.
Development styles and different Entity Framework Approaches
Before we start looking at the various options to create the EDM, let's talk about the development styles followed by the developers. Some organizations have separate teams working on the application and the database. In such cases, the database design is done first and then the application development starts. Another reason for doing the database design first is when the application being developed is a data centric application itself. Instead, it might be possible that the application demands the creation of the conceptual domain models first. Then, based on these conceptual domain models, the database tables will be created, and the application will implement the business logic in terms of these conceptual business models. Another possibility is that we are creating an application that is highly domain-centric, and the application contains the domain models implemented as classes. The database is needed only to persist these models with all the relations. There are a range of different scenarios you might find yourself in—different approaches become appropriate according to the demands of your situation. Entity Framework provides support for all these development styles and scenarios. We know that Entity Framework operates on the EDM, and lets us create this EDM in three ways in order to cater to the different development styles:
Database First:
This is the approach that will be used with an existing database schema. In this approach, the EDM will be created from the database schema. This approach is best suited for applications that use an already existing database.
Code First:
This is the approach where all the domain models are written in the form of classes. These classes will constitute our EDM. The database schema will be created from these models. This approach is best suited for applications that are highly domain-centric and will have the domain model classes created first. The database here is needed only as a persistence mechanism for these domain models.
Model First:
This approach is very similar to the Code First approach, but in this case, we use a visual EDM designer to design our models. The database schema and the classes will be generated by this conceptual model. The model will give us the SQL statements needed to create the database, and we can use it to create our database and connect up with our application. For all practical purposes, it's the same as the Code First approach, but in this approach, we have the visual EDM designer available.
Setup EntityFramework Environment:
Before stepping into using entity framework let us create MVC Application(you can use with any type of application). Let us fallow below steps:-Open Visual Studio and Click on New Project
We are done with creating MVC Application successfully..
SETUP ENTITY FRAMEWORK 6.0
Now let us setup ENTITY FRAMEWORK 6.0
- .NET Framework 4.5/Visual Express 2013
- MS SQL Server 2008/2012 Express
Right Click on Mvc Project and fallow below steps
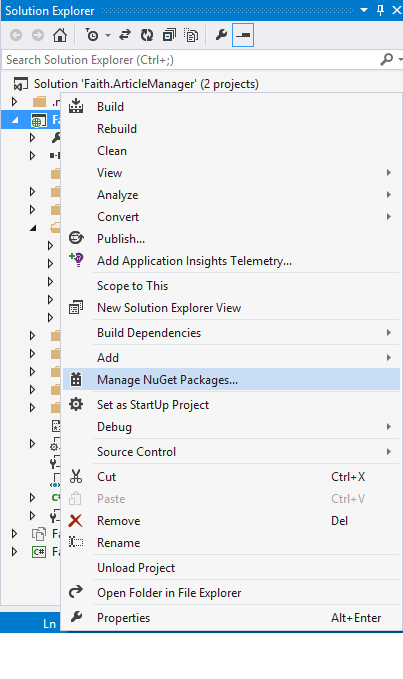
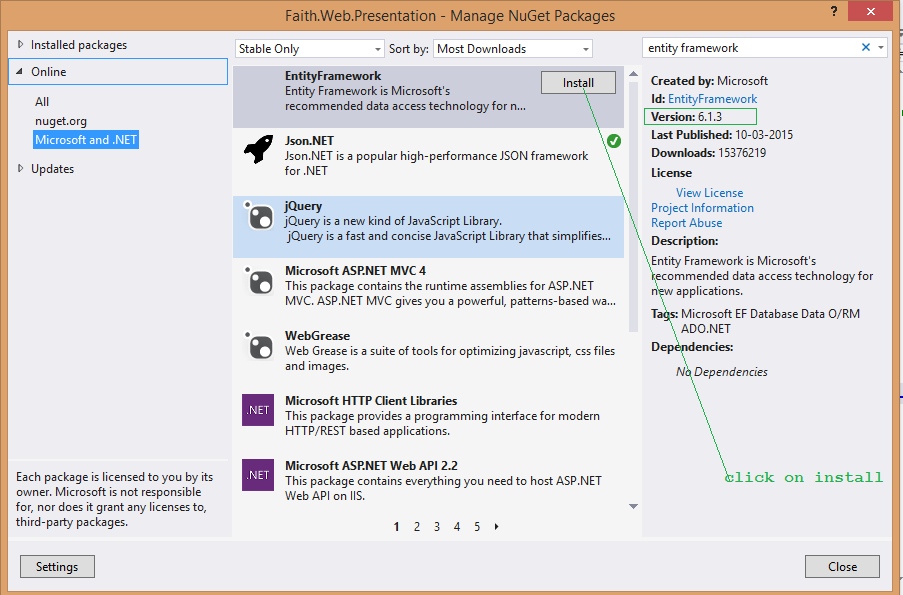
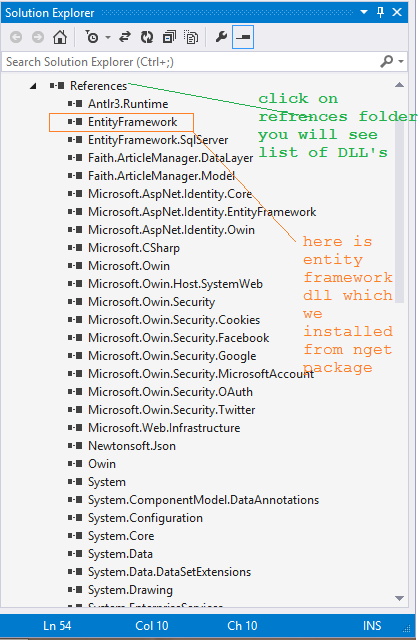
Now we have entity framework included in our project and ready to use . Now let us set up database because we are going to Database-First approach here.
Setting up the database:
Let us use sample FaithDB which has different tables, stored procedures and views. The FaithDB database design is shown below:
Here is database diagram:-
Create Entity Data Model in Entity Framework 6.0
Here, we are going to create an Entity Data Model (EDM) for FaithDb database and learn to understand basic building blocks.
You can create entity data model for existing database in Entity Framework 6.0 the same way as you created it in Entity Framework 4.1/4.3.
Let’s do it step by step.I assume here to create model as seperate project. Let us add class library like shown below.default class1.cs will get created with project creation,just remove that class.
We are done with model also just click on project ana build.
CREATE SAMPLE PROJECT ARTICLE GALLARY
Let us create a sample project where we will ADD EDIT DELETE ,SEARCH,VIEW ARTICLES, VIEW ARTICLE DETAILS using existing entity model.
We will be adding four methods to the console application:SelectArticles addArticle(), deleteArticle() and modifyArticle()
Add Controller to existing MVC application
Go to controller folder and right click on it. after right click,you can fallow below steps
In order to add new objects to the Entity Set, you must create an instance of an Entity type and add the object to the object context. Objects attached to the object context are managed by that object context. Now there are multiple ways to add a new object to the object context:
Observe the Controller code shown below:
public class ArticleController : Controller { private FaithDbEntities _dbContext = new FaithDbEntities(); public Article CurrentArticle { get; set; } // GET: /Article/ public ActionResult ArticleHome() { return View(); } [HttpPost] public ActionResult CreateArticle(Article entry) { entry.ArticlePubsDate = DateTime.Now; entry.AuthorId = 1;//owner always will change in next iteration _dbContext.Articles.Add(entry); _dbContext.ArticleAuthors.Include("AuthorId"); _dbContext.SaveChanges(); return View("Created"); } public ActionResult CreateArticle() { var articleCategoryData = _dbContext.ArticleCategories.ToList(); ViewData["_ArticleCategory"] = new SelectList(articleCategoryData, // items "ArticleCategoryId", // dataValueField "ArticleCategory1", // dataTextField 0); return View(); } [HttpPost] public ActionResult EditArticle(Article entry) { if (ModelState.IsValid) { //_dbContext.Entry(entry).State = EntityState.Modified; _dbContext.SaveChanges(); return RedirectToAction("Index"); } return View(entry); } public ActionResult EditArticle(int id) { Article article = _dbContext.Articles.Single(d => d.ArticleId == id); if (article == null) { return HttpNotFound(); } var selectedvalue = article.ArticleCategoryId; var articleCategoryData = _dbContext.ArticleCategories.ToList(); ViewData["_ArticleCategory"] = new SelectList(articleCategoryData, // items "ArticleCategoryId", // dataValueField "ArticleCategory1", // dataTextField selectedvalue); ViewData["Article"] = article; return View(article); } public ActionResult ViewArticles() { List<Article> ArtLst = new List<Article>(); using (var context = new Faith.ArticleManager.Model.FaithDbEntities()) { return View(context.Articles.ToList()); } } public ActionResult ViewArticleDetail(int id) { CurrentArticle = _dbContext.Articles.Single(d => d.ArticleId == id); return View(CurrentArticle); } public ActionResult DeleteArticle(int id) { CurrentArticle = _dbContext.Articles.Single(d => d.ArticleId == id); if (CurrentArticle == null) return View("RecordNotFound"); Delete(); _dbContext.SaveChanges(); return View("Deleted"); } [NonAction] public void Delete() { try { _dbContext.Articles.Remove(CurrentArticle); _dbContext.SaveChanges(); } catch (DataException ex) { throw ex; } } public ActionResult SearchArticle(string articleTitle, string searchText) { var ArtLst = new List<string>(); var ArtQry = from d in _dbContext.Articles orderby d.ArticleId select d.ArticleTitle; ArtLst.AddRange(ArtQry.Distinct()); ViewBag.movieGenre = new SelectList(ArtLst); var articles = from m in _dbContext.Articles select m; if (!String.IsNullOrEmpty(searchText)) { articles = articles.Where(s => s.ArticleTitle.Contains(searchText)); } if (string.IsNullOrEmpty(articleTitle)) return View(articles); else { return View(articles.Where(x => x.ArticleTitle == articleTitle)); } } public int GetAuthorIdByEmailAddress(string strEmail) { var memberId = from ArticleAuthorLogin in _dbContext.ArticleAuthorLogins where ArticleAuthorLogin.AuthorEmailId == strEmail select ArticleAuthorLogin.AuthorLoginId; return 1; } }
All we are doing here is create a new instance of the Article type and populate its properties. We then add this instance to the Articles set using the EntitySet
When context.SaveChanges() is called, EF 6.0 goes ahead and inserts the record into the database. Note that Entity Framework converts our code into queries that the database understand and handles all data interaction and low-level details.
After you have executed the code, you can go ahead and physically verify the record in the database. If the query executed successfully, you should see a new record in the Articles table of the FaithDb database, as shown below:
Update Objects in Entity Framework 6.0
In the code shown above, we are modifying the newly inserted record (‘ArticleID=1’) we created in the previous step and then add the ‘.net vs j2ee’ value for the ContactTitle column. When EF converts your code to a SQL query, it automatically uses parameters to avoid SQL injection attacks.
Execute the code and go ahead and check the record in the database:
Delete Objects in Entity Framework 6.0
To delete an existing record, retrieve an instance of the entity from the EntitySet<T> (in our case ObjectSet<Article>), then call the ObjectSet<TEntity>.DeleteObject() . When the DeleteObject() method is called, the EntityState of the object is set to ‘Deleted’
Finally call SaveChanges() on the context to delete the data from the data source. Another way to delete an object is to use the ObjectContext.DeleteObject() but we have used the ObjectSet.DeleteObject for our example
Go ahead and check the database and you will find that the ArticleID=1 no more exists.
Well this was an overview of how we can Add, Delete and Update Objects in Entity Framework 6.0.